C语言进阶-结构化的C程序设计
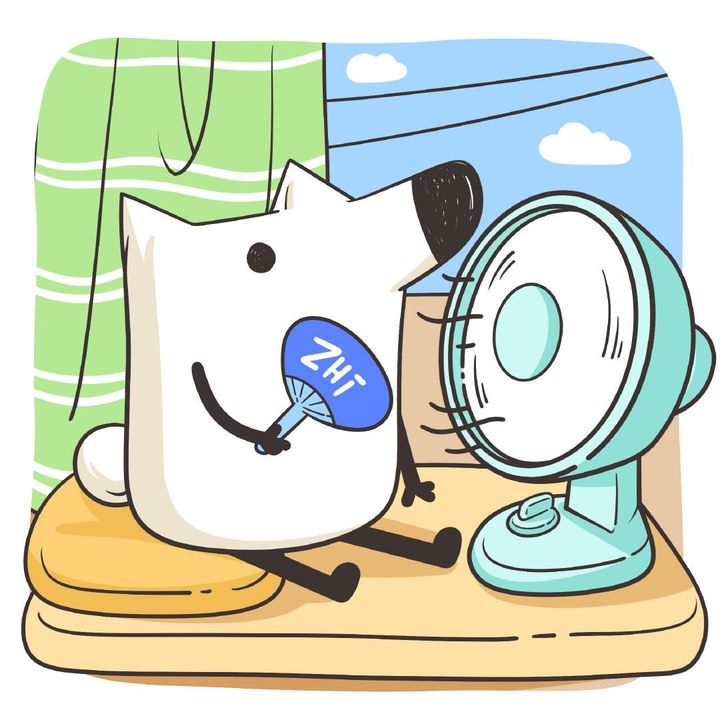
课上代码
fig3_06
1 | /*程序名:fig03_06.c*/ |
fig03_8
1 | /*程序名:fig03_08.c*/ |
fig03_10
1 | /*程序名:fig03_10.c*/ |
fig03_13
1 | /*程序名:fig03_13.c*/ |
知识点回顾:C++ 表示将 “C” 备份并作为当前参数使用,”C+1”留着下次使用。++C 表示将 “C+1” 作为当前参数使用。
课后练习
3.17
1 | /*程序名:3.17.c*/ |
3.18
1 | /*程序名:3.18.c*/ |
3.19
1 | /*程序名:3.19.c*/ |
3.20
1 | /*程序名:3.20.c*/ |
3.21
1 | /*程序名:3.21.c*/ |
3.22
1 | /*程序名:3.22.c*/ |
3.23
1 | /*程序名:3.23.c*/ |
3.24
读入10个数字并判断输出最大:
变量:
counter = 10(计数器)
number(当前输入数)
largest(当前最大数)
伪代码:
1、第一次输入值传入largest
循环部分
2、第二到十次输入值传入number
3、判断largest和number哪个值大,如果 number>largest 将number值传给largest
3.25
1 | /*程序名:3.25.c*/ |
3.26
1 | /*程序名:3.26.c*/ |
3.27
1 | /*程序名:3.27.c*/ |
3.28
1 | /*程序名:3.28.c*/ |
3.29
1 | /*程序名:3.29.c*/ |
3.30
1 | /*程序名:3.30.c*/ |
3.31
3.31a
1 | /*程序名:3.31a.c*/ |
3.31b
1 | /*程序名:3.31b.c*/ |
3.32
3.32a
1 | /*程序名:3.32a.c*/ |
3.32b
1 | /*程序名:3.32b.c*/ |
3.32c
1 | /*程序名:3.32c.c*/ |
3.32d
1 | /*程序名:3.32d.c*/ |
3.33
1 | /*程序名:3.33.c*/ |
3.34
1 | /*程序名:3.34.c*/ |
3.35
1 | /*程序名:3.35.c*/ |
3.36
1 | /*程序名:3.36.c*/ |
3.37
1 | /*程序名:3.37.c*/ |
3.38
1 | /*程序名:3.38.c*/ |
3.39
1 | /*程序名:3.39.c*/ |
3.40
1 | /*程序名:3.40.c*/ |
3.41
1 | /*程序名:3.41.c*/ |
3.42
1 | /*程序名:3.42.c*/ |
3.43
1 | /*程序名:3.43.c*/ |
3.44
1 | /*程序名:3.44.c*/ |
3.45
1 | /*程序名:3.45.c*/ |
3.46
1 | /*程序名:3.46.c*/ |
3.47
3.47a
递归算法
1 | /*程序名:3.47a.c*/ |
循环
1 | /*程序名:3.47a.c*/ |
3.47b
1 | /*程序名:3.47b.c*/ |
3.47c
1 | /*程序名:3.47c.c*/ |
- 本文标题:C语言进阶-结构化的C程序设计
- 本文作者:9unk
- 创建时间:2023-09-10 18:18:00
- 本文链接:https://9unkk.github.io/2023/09/10/c-yu-yan-jin-jie-jie-gou-hua-de-c-cheng-xu-she-ji/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!